Polar Codes
This package provides the Polar Encoder and Polar Decoder build on the top of Sionna modules to provide low level 3GPP 5G-NR standards complaint API which can be used with all the uplink and downlink channel. The Polar coders can be easily integrated with rate matching, code block segmentation and concatenation. Polar coders are used by broadcast and control channels for conveying small payload size over the wireless channels. 5G uses Polar codes for following chains where A, N and E are polar encoder input length, mother codeword length and Payload length respectively:
Physical Chains |
\(\text{I}_{SEG}\) |
\(\text{I}_{IL}\) |
\(\text{I}_{BIL}\) |
A |
N |
E |
---|---|---|---|---|---|---|
PBCH |
0 |
1 |
0 |
32+24 |
512 |
864 |
PDCCH |
0 |
1 |
0 |
1 to 140 |
32 to 512 |
25 to 8192 |
PUCCH-Format3 |
0 |
0 |
1 |
12 to 1706 |
32 to 1024 |
18 to 8192 |
PUCCH-Format4 |
1 |
0 |
1 |
12 to 1706 |
32 to 1024 |
31 to 16384 |
Important
Polar code is used in 5G for control and broadcast information in uplink and downlink channel which generally has has small payload size and require very high reliability.
The reliability results for Polar codes are shown in following figure-1 where bit error rate results are plotted against signal to noise ratio (SNR) for various block-lengths relevant to downlink control channel.
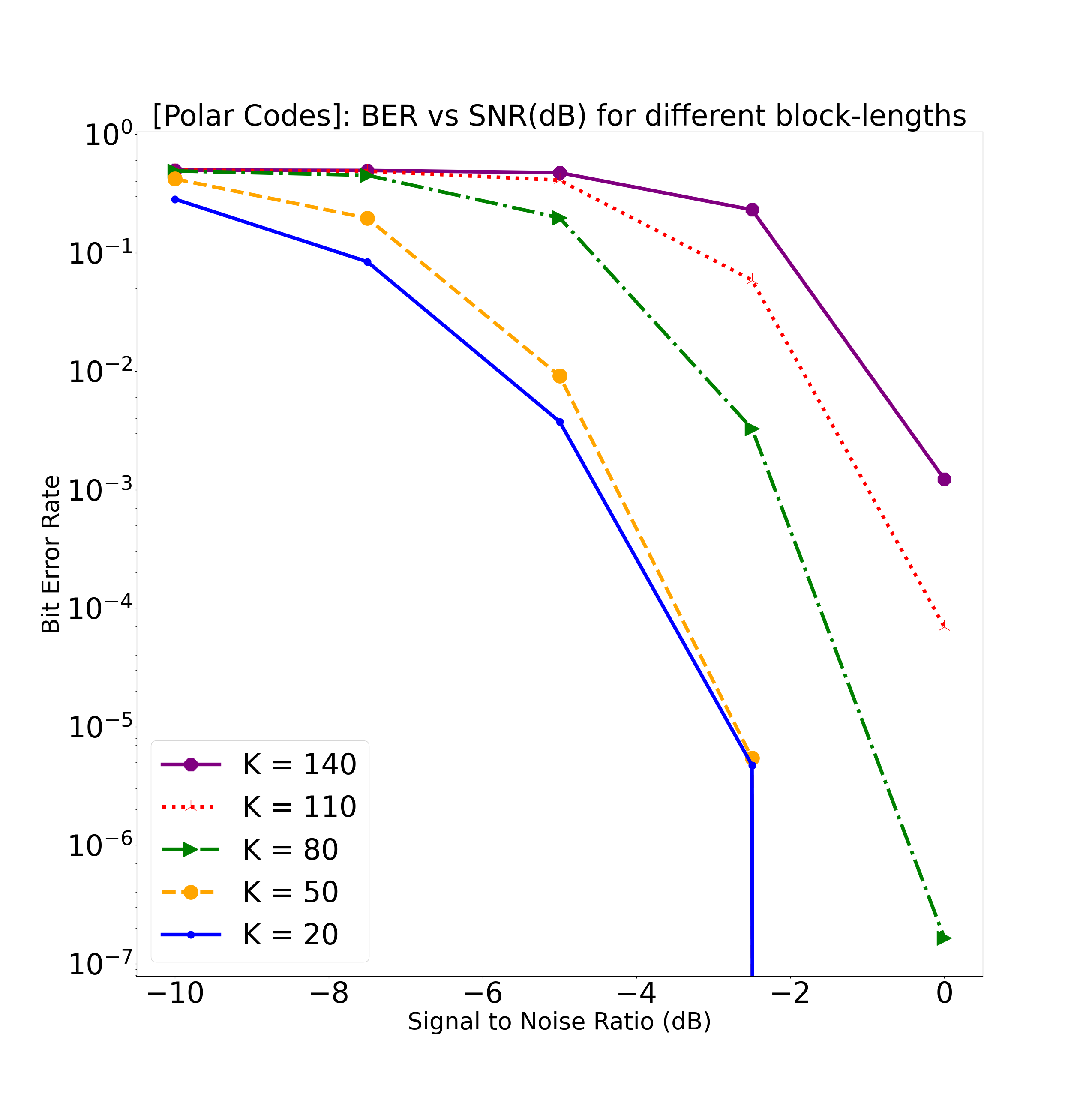
Polar Encoder
The details of Polar Encoder is provided in section 5.3.1 of [3GPPTS38212Polar]. There are few examples to illustrate how to use Polar Encoder for simulations:
nBatches= 5 # Number of Batches to Encode
K = 56 # Number of bits to encode
E = 864 # Rate matching bits
purpose = "PDCCH" # The channel for which the Polar Encoder | Dimension = (numBatches, K)
verbose = False
# Bits to encode
bits = np.float32(np.random.randint(2, size=(nBatches, K)))
# Create Object for Polar Encoding
encoder = PolarEncoder5G(K, E, purpose,verbose)
codeword= encoder(bits) # Generate Encoded Bits using the Encoder Object | Dimension = (numBatches, N)
print("N: "+str(encoder.N))
The details about the input-output interface of the PolarEncoder module is provided below.
- class toolkit5G.ChannelCoder.PolarEncoder5G(K, E, purpose, verbose)[source]
This module implements a 5G standards complaint Encoder for Polar Codes. The modules is built on the top on Sionna Polar Decoder with modifications to provide low level API for section 5.3.1 of 38.212 specifications [3GPPTS38212Polar]. Moreover, it corrects the broken standards complaince and optimize the implementation for performance and memory.
- Parameters:
K (int) – Number of information bits to encode.
E – Number of target bits after rate matching.
purpose (str) – Defines the physical channel for which the Polar Decoder is being used. It is used computation of N (number of coded bits at the out of Polar Decoder) \(\in \{\) “PBCH”, “PDCCH”, “PUCCH” \(\}\).
verbose (bool) – Defaults to False. If True, rate-matching parameters will be printed.
- Input:
inputs ([…,K], tf.float32) – 2+D tensor containing the ‘K’ information bits to be polar encoded.
- Output:
[…,N], np.float32 – 2+D tensor containing decoded N encoded information bits.
- Raises:
Exception – [Error]: Invalid value for “”K”” for UCI:PUCCH!
Exception – [Error]: Invalid value for “”purpose””!
ValueError – [Polar Encoder]: ‘input’ must a numpy array of numbers!
Note
Although the decoding list size is not provided by 3GPP [3GPPTS38212Polar], the consortium has agreed on a list size of 8 for the 5G decoding reference curves [Bioglio].
All list-decoders apply CRC-aided decoding, however, the non-list decoders (“SC” and “BP”) cannot materialize the CRC leading to an effective rate-loss.
- property N
Defines the Codeword length of mother Polar code. Calculated based on Section 5.3.1 of TS 38.212.
Polar Decoder
The Polar decoder can be implemented using multiple methods and each method provides a trades off between accuracy and complexity as shown below.
Method |
Parameters |
Complexity |
Performance |
---|---|---|---|
“SC” |
– |
Low (1) |
Poor (1) |
“SCL” |
list_size |
High (2) |
Good (2) |
“hybSCL” |
list_size |
Highest (3) |
Best (3) |
“BP” |
num_iter |
Lowest (0) |
Poorest (0) |
which uses implementations provided by Sionna.
logits = 100*(2*codeword-1) # Logits/LLR to decode the bits | Dimension = (numBatches, N)
K = 56 # Number of bits to encode
E = 864 # Rate matching bits
dec_type = 'SC' # Polar Decoder Type is "Successive Cancellation type".
crcType = 'CRC24C' # CRC used for error detection (not relevant in this case.)
purpose = 'PDCCH' # Physical channel for whose to decode the payload.
list_size = 8 # List Size (not relevant in this case.)
num_iter = 20 # Number of iterations (not relevant in this case.)
# Create Object for Polar Decoder
decoder = PolarDecoder5G(K = K, E = E, purpose = purpose)
bitEst = decoder(logits) # Recover information Bits using the Decoder Object | Dimension = (numBatches, K)
- class toolkit5G.ChannelCoder.PolarDecoder5G(K, E, dec_type='SC', crcType='CRC24C', purpose='PDCCH', list_size=8, num_iter=20, output_dtype=tf.float32, **kwargs)[source]
This module implements a 5G standards complaint decoder for Polar Codes. The modules is built on the top on Sionna Polar Decoder with modifications to provide low level API for section 5.3.1 of 38.212 specifications [3GPPTS38212Polar]. Moreover, it corrects the broken standards complaince and optimize the implementation for performance and memory. The class inherits from the Keras layer class and can be used as layer in a Keras model.
- Parameters:
K (int) – Number of information bits to encode.
E – Number of target bits after rate matching.
dec_type (str) – Polar Decoder type. It can be \(\in \{\) “SC”, “SCL”, “hybSCL”, “BP” \(\}\). Default value is “SC”.
crcType (str) – CRC Type used error detection. It is used for hybrid SCL decoding. \(\in \{\) “CRC24A”, “CRC24B”, “CRC24C”, “CRC16”, “CRC11”, “CRC6” \(\}\). Default value is “CRC24C”. Only required for
dec_types
is “SCL”.purpose (str) – Defines the physical channel for which the Polar Decoder is being used. It is used computation of N (number of coded bits at the out of Polar Decoder) \(\in \{\) “PBCH”, “PDCCH”, “PUCCH” \(\}\). Default value is “PDCCH”.
list_size (int) – Defining the list size iff list-decoding is used. Only required for
dec_types
{“SCL”, “hybSCL”}. Default value is 8.num_iter (int) – Defining the number of BP iterations. Only required for dec_type “BP”. Default value is 20.
output_dtype (tf.DType) – Defaults to tf.float32. Defines the output datatype of the layer (internal precision remains tf.float32).
- Input:
inputs ([…,N], np.float32) – 2+D tensor containing the ‘N’ ratematched llr logits/llr values.
Warning
Polar Decoder doesn’t accept binary inputs. In case only hard information is available, convert the bits to logits: \(2\times\text{inputs}-1\) and pass it to Polar Decoder.
- Output:
[…,K], tf.float32 – 2+D tensor containing hard-decoded estimates of all k information bits.
- Raises:
ValueError – “[Polar Decoder]: Invalid
crcType
!”ValueError – “[Polar Decoder]: Unknown value for
dec_type
.”ValueError – “[Polar Decoder]: Invalid input dtype!”
Note
Although the decoding list size is not provided by 3GPP [3GPPTS38212Polar], the consortium has agreed on a list size of 8 for the 5G decoding reference curves [Bioglio].
All list-decoders apply CRC-aided decoding, however, the non-list decoders (“SC” and “BP”) cannot materialize the CRC leading to an effective rate-loss.
- property E
Number of rate matched bits (target Bits, Defined as E in Section 5.3.1 of TS 38.212).
- property K
Number of information bits passed as returned by Polar Decoder (mother Polar code).
- property N
Defines the Codeword length of mother Polar code. Calculated based on Section 5.3.1 of TS 38.212.
- property dec_type
Defines the Decoder type used for decoding the codeword. Its a string type
- property llr_max
Defines the Maximum LLR value for internal calculations. Its the saturation value of LLR. Its afloat type
- property output_dtype
Output dtype of decoder.
Polar Codec Components
The Polar Codec in 5G consists of multiple other components to implement the complete physical chain. These modules are discussed in following subsections: