PDCCH Scheduler
The class PDCCHScheduler
implements the functionality of base station (BS) scheduling PDCCH to a certain number of UEs in a given slot. [3GPPTS38213PDCCH]
Scheduler allocates resources to each UE in a cell. One key system performance evaluation metric is the PDCCH blocking probability which indicates the percentage of user equipments
(UEs) that cannot be scheduled by the Base Station for receiving the DCI.
Given the number of UEs and the coverage condition, Base Station can properly determine the CORESET size (i.e, number of Control Channel Elements or CCEs) to ensure the blocking probability does not exceed a specified threshold.
The Base Station (BS) can use different PDCCH candidates with in the CORESET for scheduling different UEs. Blocking occurs for a UE when there are no non-overlapping candidates available for scheduling.
The variable numCCEs
correspond to CORESET size and numCandidatesUnderEachAL
captures the number of PDCCH candiates per Aggregation Level (AL).
The position of different PDCCH candidates for each AL is determined using a hashing equation. For more information please refer to section 10.1 of 3GPP TS 38.213.
- class toolkit5G.Scheduler.PDCCHScheduler(mu, slotNumber, coresetID, nci=0)[source]
A class which implements Physical Downlink Control Channel (PDCCH) scheduler.
- Parameters:
mu (int) – Specifies the numerology or sub-carrier spacing (SCS) in 5G-NR. Must be a positive int and defaults to 0. i.e., with a SCS of 15 KHz. Supported numerology are {0,1,2,3}, which correspond to a SCS of {15,30,60,120} KHz respectively.
slotNumber (int) – Defines the slot number within a frame where PDCCH is loadedS \(n_{s,f}^{\mu} \in \{0,1,2,\) \(\dots,10*2^{\mu}-1\}\) where \({\mu = \frac{\Delta f}{15000}}\).
coresetID (int) – Specifies the ID of CORESET configured in a BWP. Can be either 1 or 2 or 3. For more information refer variable p from section 10.1 of 3GPP TS 138.213.
nci (int) – Defines the variable corresponding to carrier aggregation (CA) in computation of cce indices. For more information refer section 10.1 of 3GPP TS 38.213. Current implementation does not support CA and hence defaults to 0.
- Input:
numCCEs (int) – Defines the CORESET size. Typically expressed in terms of number of Control Channel Elements (CCEs) available for scheduling the UEs. Must be a positive int. Scheduler has to choose this value depending on the number of UEs to be scheduled and the aggregation levels that it has to support for each UE. See the note below for more information.
searchSpaceType (str) – Specifies the type of search space set. Must be a string. Valid inputs are {CSS,USS}, where CSS denote Common Search Space Set and USS denote UE Specific Search Space Set respectively. Valid Aggregation Levels (ALs) when search space set is USS are {1,2,4,8,16} and {4,8,16} for CSS respectively. For more information on search space set refer section 10.1 of 3GPP TS 38.213, UE procedure for determining physical downlink control channel assignment.
UEsUnderEachAL (np.ndarray of int of size 5.) –
Defines the distribution of number of UEs under each aggregation level (AL).
First int correspond to number of UEs under AL 1, followed by number of UEs under AL 2, and likewise till number of UEs under AL 16.
For instance [5,2,1,4,3] correspond to 5 UEs taking AL 1, 2 UEs of AL 2, 1 UE under AL 4, 4 UEs of AL 8 and 3 UEs of AL 16 respectively for a total number of UEs to schedule is 15.
numCandidatesUnderEachAL (np.ndarray of int of size 5.) –
Defines the distribution of number of pdcch candidates under each aggregation level (AL) for each UE.
\(L\) consecutive CCEs forms a pdcch candidate for a given aggregation level \(L\).
For instance [6,6,4,2,1] correspond to 6 AL 1 candidates, 6 AL 2 candidates, 4 AL 4 candidates, 2 AL 8 candidates and 1 AL 16 candidate respectively.
rnti (int) – It defines the radio network temporary identifier (RNTI) \(\in \{1,2,..,65519\}\). If the search space set is USS, then the allocated cce indices for a chosen aggregation level depends on the RNTI value, whereas for CSS they are independent.
strategy (str) –
Defines the scheduling strategy.
Supported strategies are “Conservative”, “Aggressive”, and “Random”.
“Conservative” correspond to scheduler prioritize UEs which take lower ALs.(i.e., UEs with low ALs are scheduled first)
“Aggressive” correspond to scheduler prioritize UEs which take higher ALs (i.e., UEs with high ALs are scheduled first)
“Random” correspond to scheduler prioritize UEs at random that can take any AL.
Defaults to “Conservative”.
- Output:
tuple of two np.ndarrays each having a dimension 5 or 3 depending on the search space set associated with a coreset. For USS they are 5 dimensional and for CSS they are 3 dimensional. –
First element of the tuple correspond to an array of dimenison 5 or 3, denoting the number of blocked UEs under each AL for a given coreset size
numCCEs
andUEsUnderEachAL
. Blocked UEs correspond to the number of UEs which are not successfully scheduled by a base station (BS) in the current PDCCH opportunity. These UEs need to be scheduled by the BS at another PDCCH opportunity.Second element of the tuple correspond to an array also of dimenison 5 or 3 depending on the search space set it is associated with.
Each element in this array itself is a numpy array of shape (number of UEs under a particular AL, 2). For instance ith element has the shape (UEsUnderEachAL[i], 2)
The number 2 at last axis captures the first and last cce index (as they are allocated continuously by the scheduler) of each UE under AL \(i\) respectively.
If UEsUnderEachAL[i] = 0, then correspondingly -1 is returned as a cce index.
For each UE under AL of 1, the first and last CCE index are same as AL of 1 would require only 1 CCE to schedule.
see the note below for more understanding.
- Raises:
ValueError – If
searchSpaceType
is not a common search space “CSS” set or a UE specific search space “USS” set.ValueError – If
UEsUnderEachAL
is not a numpy array of 5 positive integers specifying the number of UEs under each AL.ValueError – If
numCandidatesUnderEachAL
is not an array of 5 positive integers specifying the number of PDCCH candidates under each AL.ValueError – If
strategy
is not a string taking values other than from the set {Conservative, Aggressive, Random}.
Note
Example to understand the output format.
For instance if the number of UEs to schedule are 5 with an AL distribution of [0.4, 0.3, 0.2, 0.05, 0.05] resulting in
UEsUnderEachAL
of [2 1 1 0 1] produce the following output assuming USS:(array([0, 0, 0, 0, 0]), array([array([[21, 21], [12, 12]]), array([[10, 11]]), array([[24, 27]]), array([-1, -1]), array([[32, 47]])], dtype=object))
First element of the tuple correspond to a numpy array of all zeros, denoting the number of blocked UEs under each AL is 0. i.e., number of blocked UEs under AL 1 is 0, number of blocked UEs under AL 2 is 0, number of blocked UEs under AL 4 is 0, number of blocked UEs under AL 8 is 0, number of blocked UEs under AL 16 is 0 respectively.
Second element from the tuple captures cce indices of each UE under each AL starting with AL of 1 as shown below.
For i = 0, UEsUnderEachAL[i] = 2, which results in to an array of shape (2,2). i.e., array([[21, 21], [12, 12]]).
array([[21, 21], [12, 12]]) correspond to the cce indices of 2 UEs under AL 1, where 21 is the cce index allocated for the first UE under AL 1, and 12 is the cce index chosen for second UE under AL 1 respectively.
array([[10, 11]]) correspond to the cce index of 1 UE under AL 2.
array([[24, 27]]) correspond to the first and last cce index of 1 UE under AL 4.
array([-1, -1]) denotes that there are no UEs to schedule for AL 8.
array([[32, 47]]) correspond to the first and last cce index of 1 UE under AL 16.
Note
Maximum number of PRBs available in a BWP is 270.
If CORESET occupies the entire BWP and is configured with duration of 1 ofdm symbol, then max number of CCEs available are 270/6, which is 45.
If CORESET occupies the entire BWP and is configured with duration of 2 ofdm symbol, then max number of CCEs available are (270*2)/6, which is 90.
If CORESET occupies the entire BWP and is configured with duration of 3 ofdm symbol, then max number of CCEs available are (270*3)/6, which is 135.
Typically Base Station (BS) chooses any value from the above depending on the number of UEs to be scheduled at that PDCCH opportunity.
Note
Scheduler always choses higher aggregation level (typically either 8 or 16) for those UEs which have poor SNR/SINR (i.e., those UEs which are at cell edge) under extreme coverage conditions.
Moderate aggregation level (i.e, 4) for those UEs which have decent SINR under moderate coverage conditions.
Lower aggregation level (i.e, either 1 or 2) for those UEs which have good coverage condition.
Note
“Conservative” strategy has the advantage that it prioritizes UEs with lower aggregation levels that uses lower number of CCEs, thus resulting in more UEs being scheduled and a lower blocking probability..!
The following figure displays the coreset region with in a BWP, where a PDCCH can be transmitted for the example shown below.
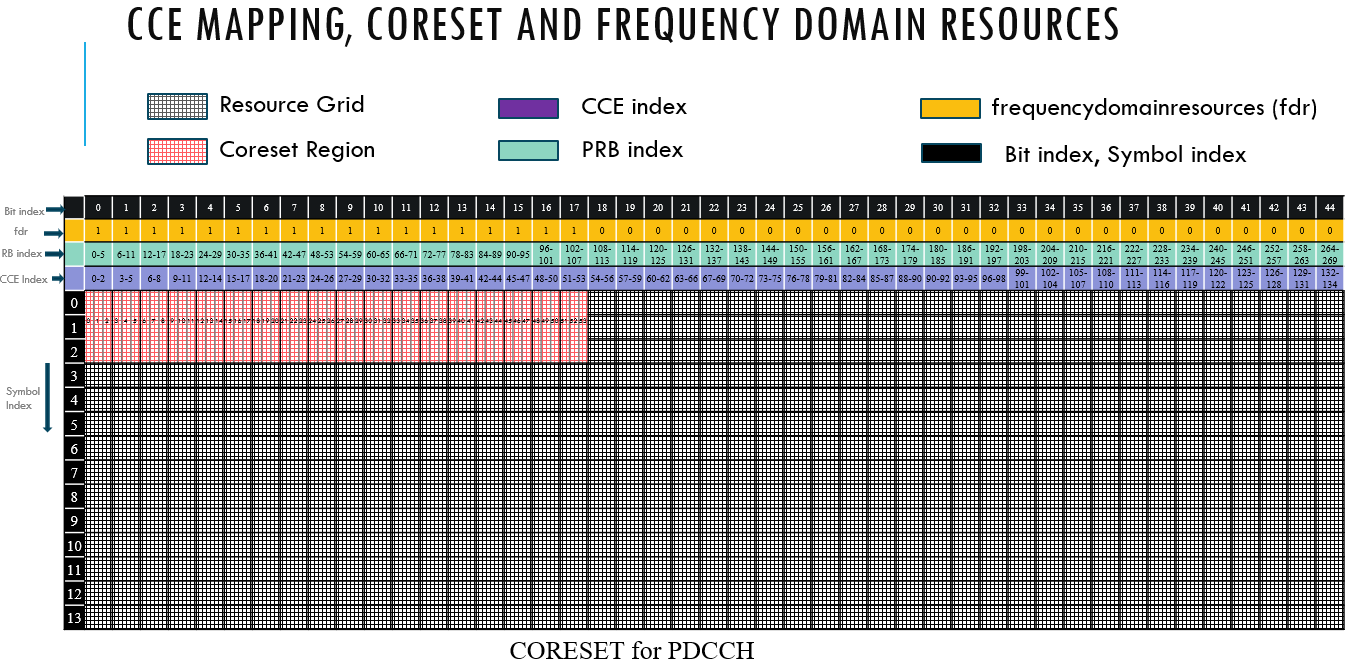
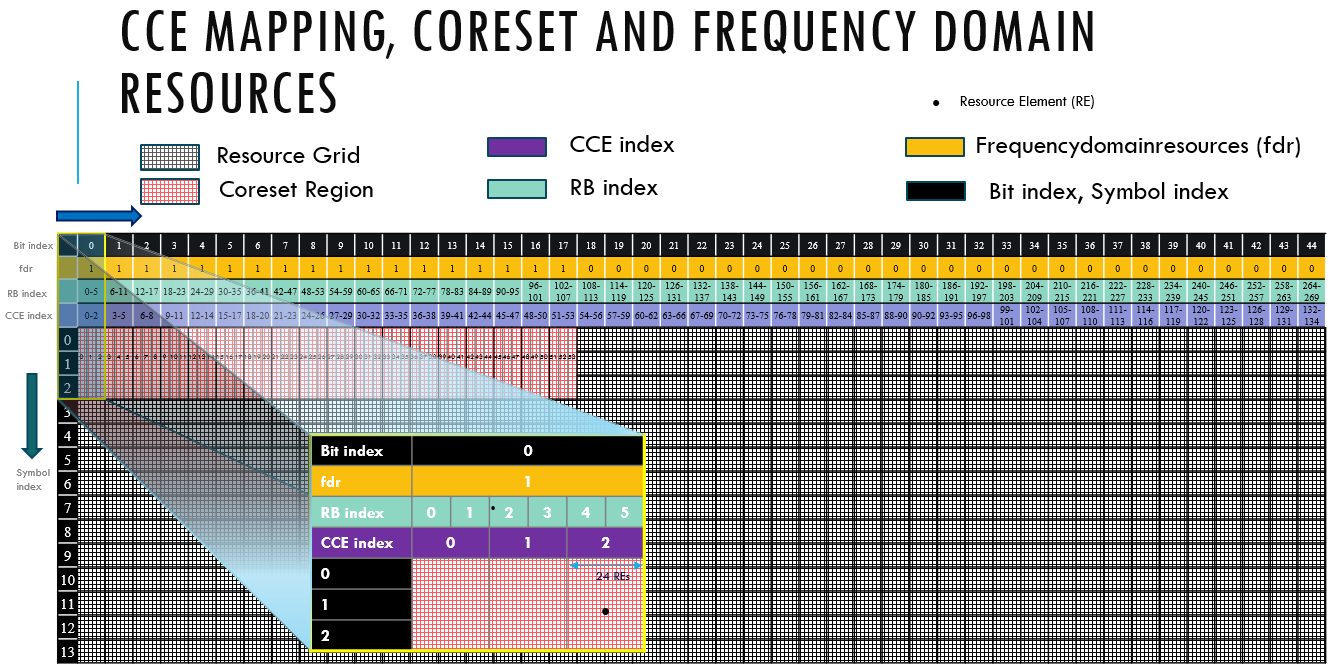
Example:
The following code snippet shows the use of PDCCH scheduler class. 5 UEs have been scheduled by the Base Station (BS) having a coreset size of 54. The probability distribution for each aggregation level considered in the simulation is [0.4, 0.3, 0.2, 0.05, 0.05], and the number of pdcch candidates under each aggregation level considered is [6,6,4,2,1] respectively.
#######################################
#Importing necessary Python Libraries
#######################################
import os
os.environ["CUDA_VISIBLE_DEVICES"] = "-1"
os.environ['TF_CPP_MIN_LOG_LEVEL'] = '3'
%matplotlib widget
import matplotlib.pyplot as plt
import matplotlib.patches as mpatches
import matplotlib as mpl
import numpy as np
############################
#Importing toolkit5G modules
############################
import toolkit5G
from toolkit5G.Scheduler import PDCCHScheduler
########################
## Simulation Parameters
########################
Nccep = 54 # Coreset size or Number of CCEs
mu = np.random.randint(4) # numerlogy for sub-carrier spacing
numSlotsPerFrame = 2**mu * 10 # number of slots per radio frame
coresetID = 1 # coreset ID
slotNumber = 0
duration = 3 # duration of coreset in number of ofdm symbol
searchSpaceType = "USS" # search space type. UE specific search space
nci = 0 # variable corresponding to carrier aggregation
############################
# PDCCH Scheduler Parameters
############################
strategy = "Conservative"
numUEs = 5
aggLevelProbDistribution = np.array([0.4, 0.3, 0.2, 0.05, 0.05]) # UE Aggregation Level Probability Distribution
numCandidatesUnderEachAL = np.array([6,6,4,2,1], dtype=int) # Number of PDCCH Candidates per Aggregation Level
UEsUnderEachAL = np.random.multinomial(numUEs, aggLevelProbDistribution)
rnti = np.random.choice( np.arange(1,65519+1), size = (numUEs,), replace=False)
print("############################################################################################")
print()
print("Number of UEs in simulation:", numUEs)
print()
print("RNTI corresponding to each UE:", rnti)
print()
print("############################################################################################")
pdcchSchedulerObj = PDCCHScheduler(mu, slotNumber, coresetID, nci)
count, cceIndices = pdcchSchedulerObj(Nccep,searchSpaceType,UEsUnderEachAL,numCandidatesUnderEachAL,rnti,strategy)
numBlockedUEs = np.sum(count)
print("############################################################################################")
print()
print("Number of UEs under each Aggregation Level:", UEsUnderEachAL)
print()
print("CCE Indices corresponding to all UEs being Scheduled:\n", cceIndices)
print()
print("Number of Blocked UEs:", numBlockedUEs)
print("############################################################################################")
print()
print("Available PDCCH Candidates for scheduler:\n", pdcchSchedulerObj.candidates)
############################################################################################
Number of UEs in simulation: 5
RNTI corresponding to each UE: [14766 34333 8673 37708 57818]
############################################################################################
############################################################################################
Number of UEs under each Aggregation Level: [2 1 1 0 1]
CCE Indices corresponding to all the UEs being Scheduled:
[array([[51, 51],
[ 4, 4]]) array([[22, 23]]) array([[16, 19]]) array([-1, -1])
array([[ 0, 15]])]
Number of Blocked UEs: 1
############################################################################################
Available PDCCH Candidates for scheduler:
array([array([[ [51],
[ 6],
[15],
[24],
[33],
[42]],
[ [ 4],
[13],
[22],
[31],
[40],
[49]]]), array([[[22, 23],
[30, 31],
[40, 41],
[48, 49],
[ 4, 5],
[12, 13]]]), array([[[ 4, 5, 6, 7],
[16, 17, 18, 19],
[28, 29, 30, 31],
[44, 45, 46, 47]]]),
array([], shape=(0, 2, 8), dtype=int64),
array([[[ 0, 1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11, 12, 13, 14, 15]]])],
dtype=object)
The following figure displays the allocated PDCCH candidates in terms of CCE indices for each UE and for all the possible aggregation level. All the occupied or unavailable candidates are marked as x.
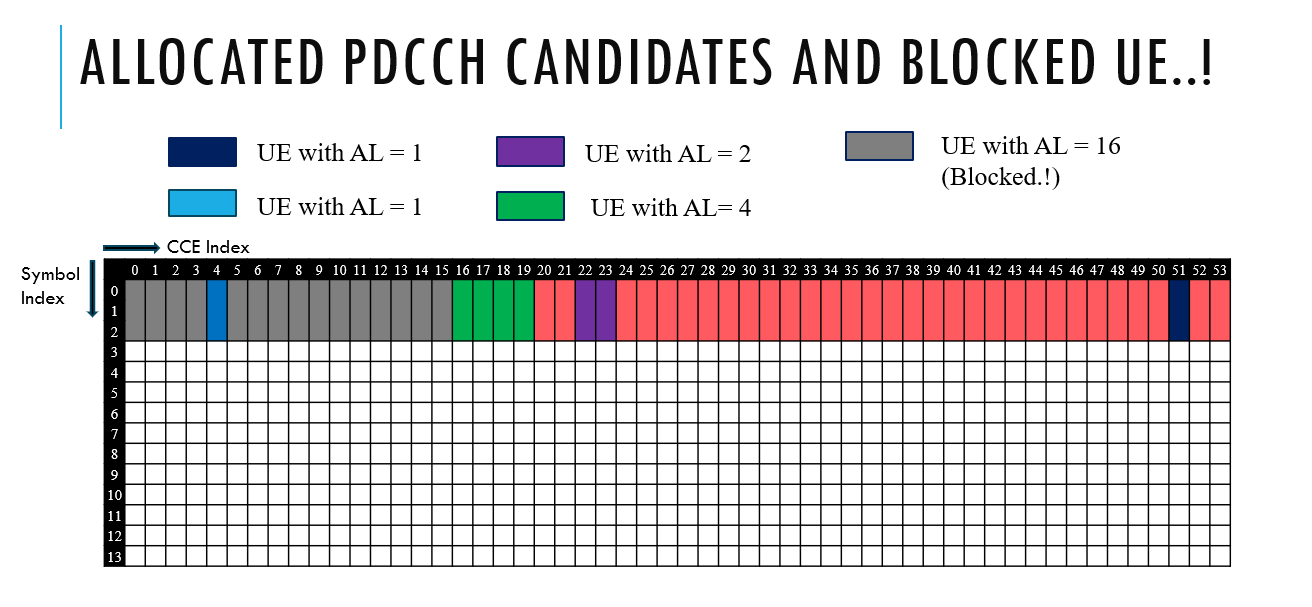
The cce indices chosen for UEs with AL=1 is shown below:
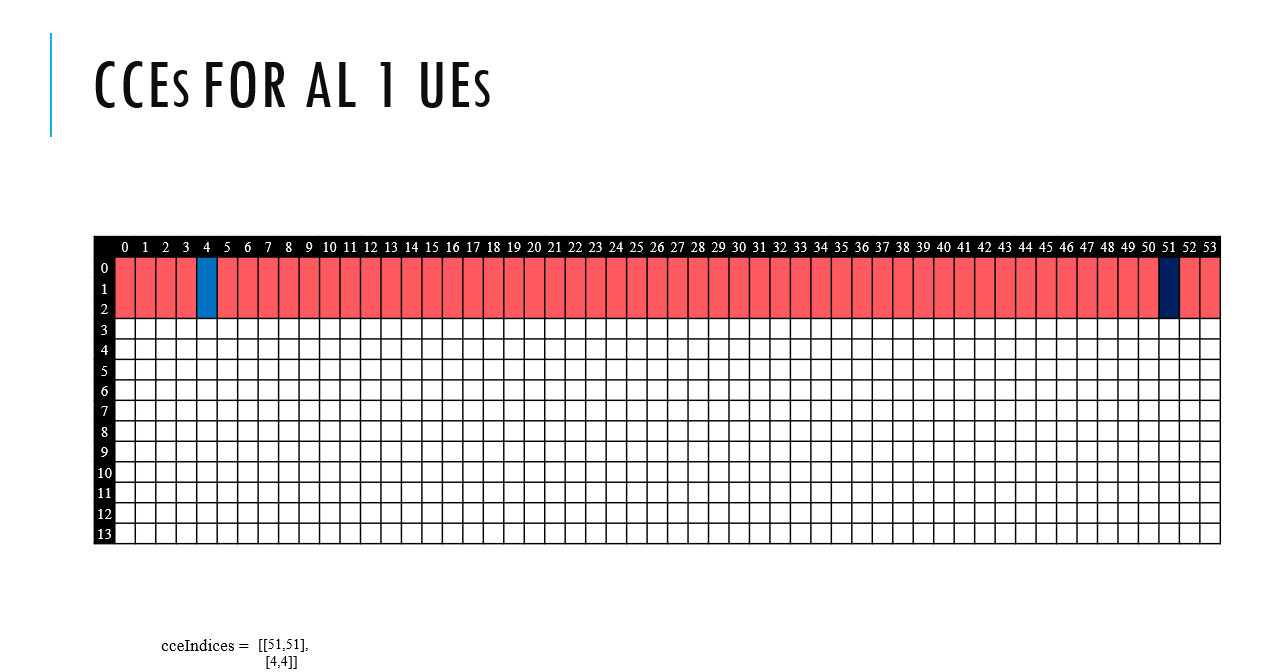
The cce indices chosen for UEs with AL=2 is shown below:
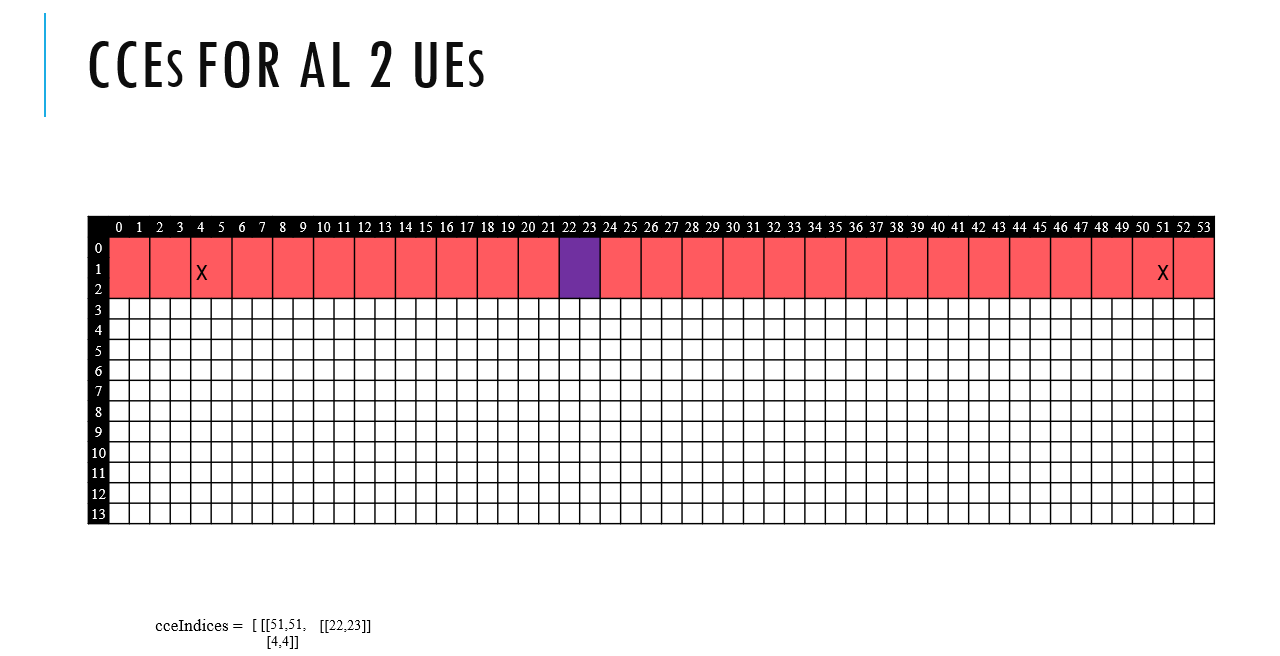
The cce indices chosen for UEs with AL=4 is shown below:
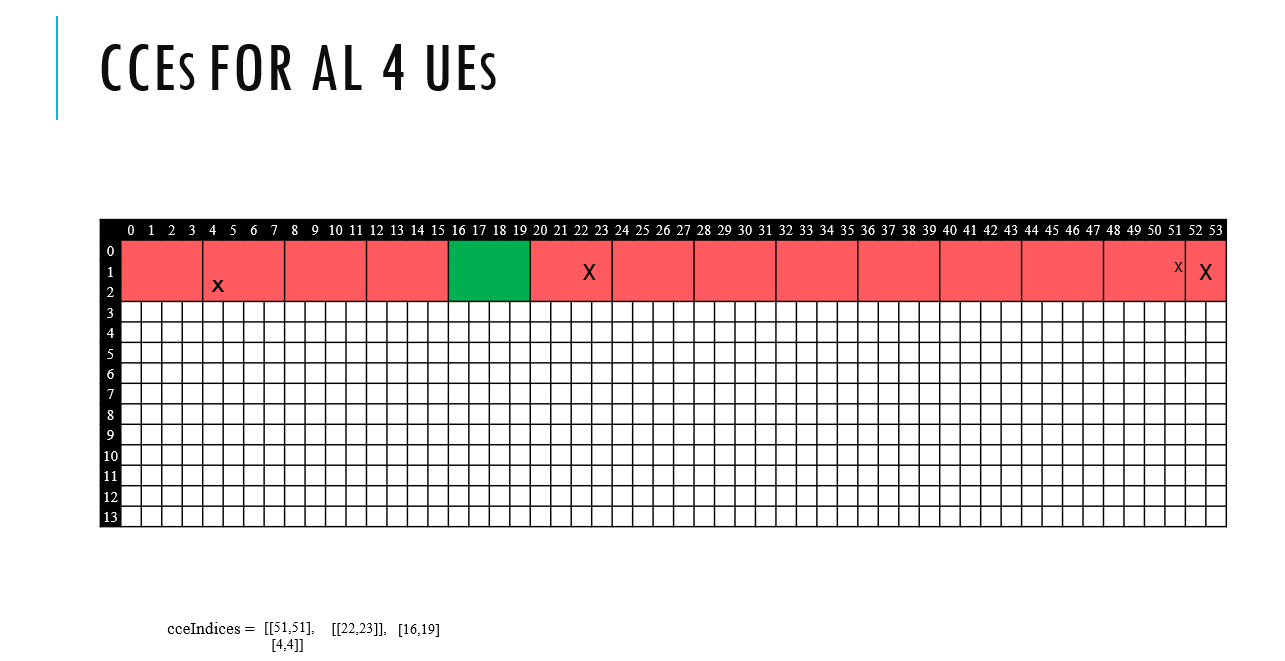
The cce indices chosen for UEs with AL=8 is shown below:
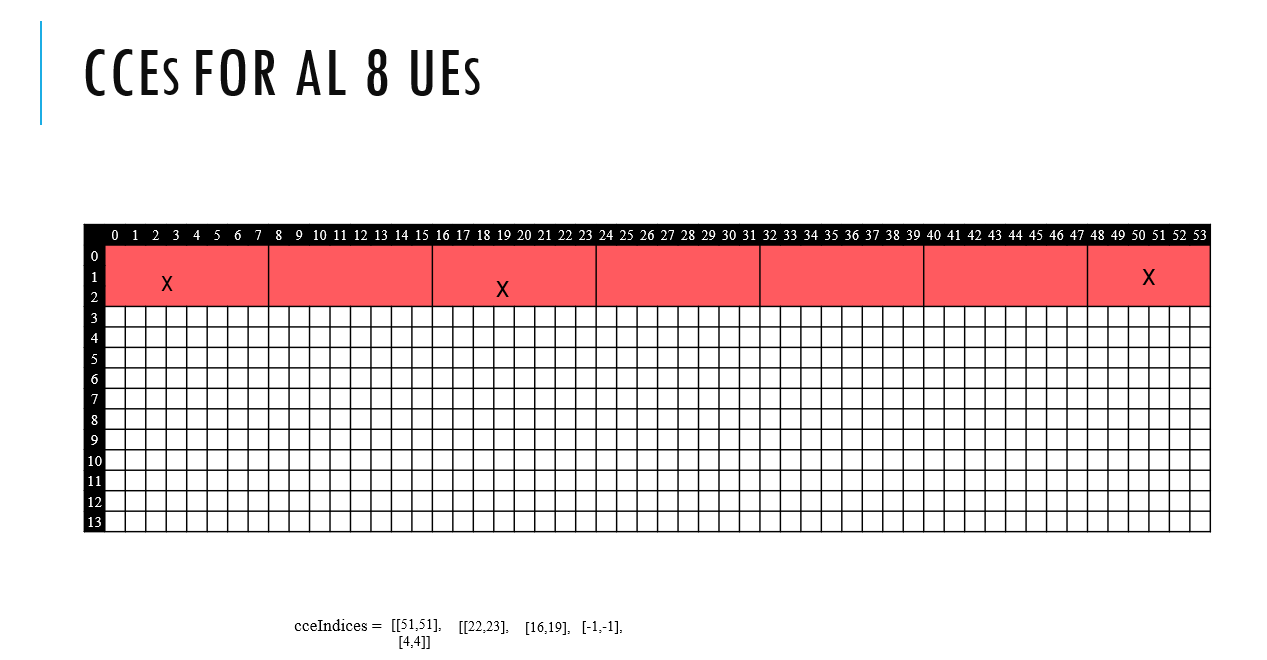
The cce indices chosen for UEs with AL=16 is shown below:
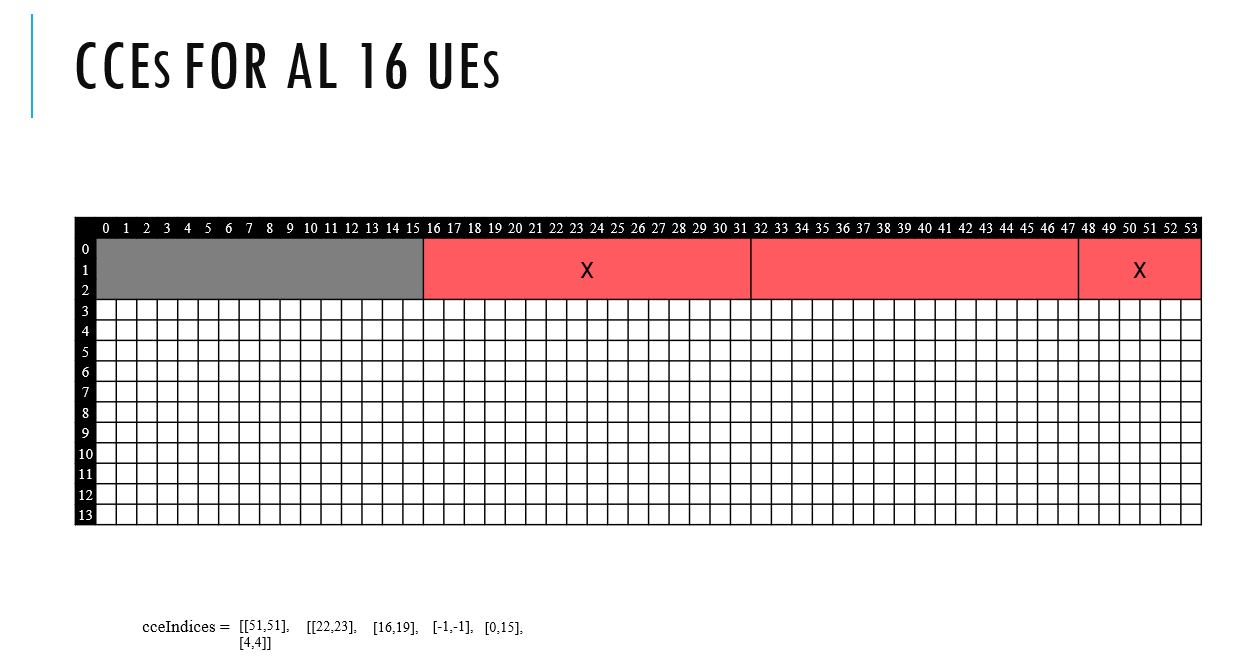
The PDCCH candidates available in the CORESET region, and the chosen candidates by the scheduler is also shown below. The following figure shows the available AL 1 candidates.
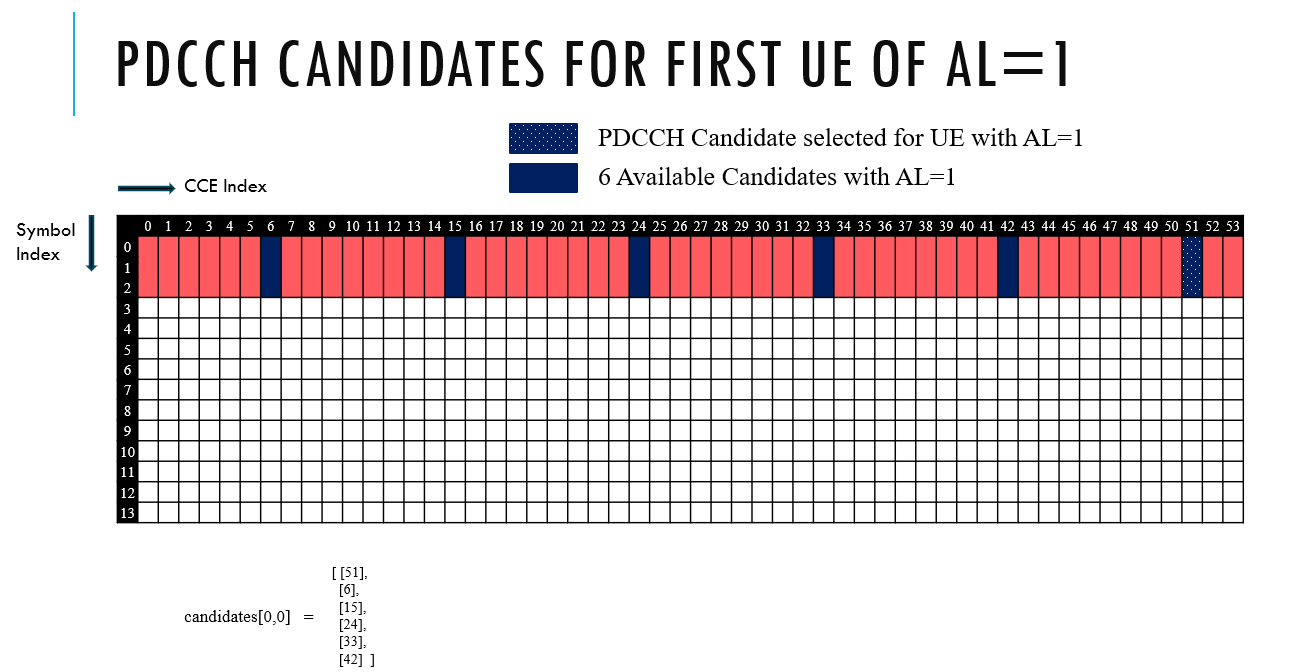
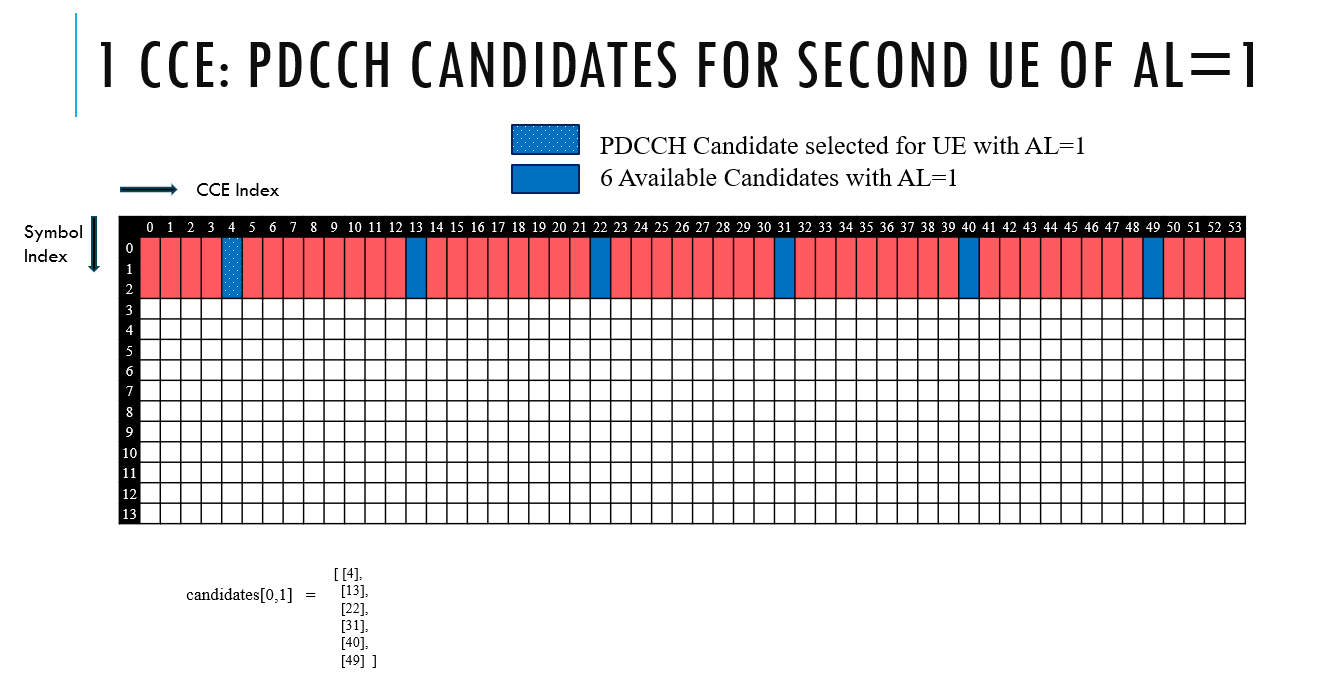
AL 2 candidates are shown below:
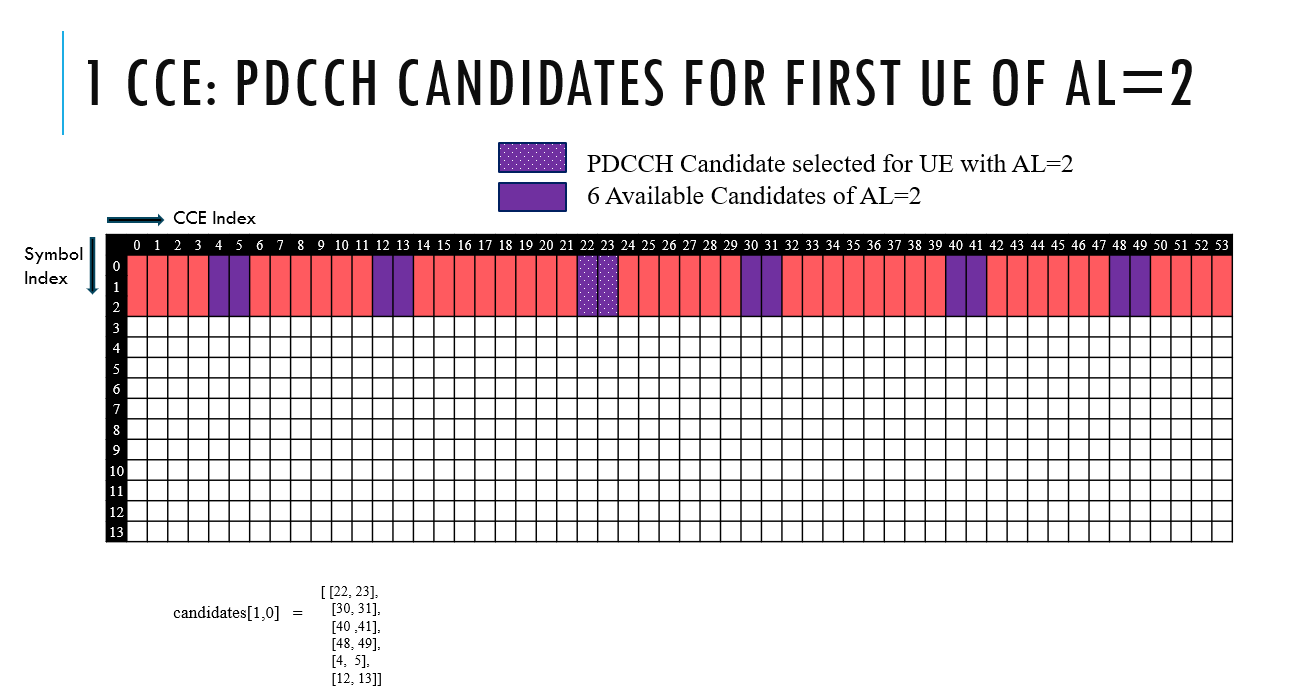
AL 4 candidates are shown below:
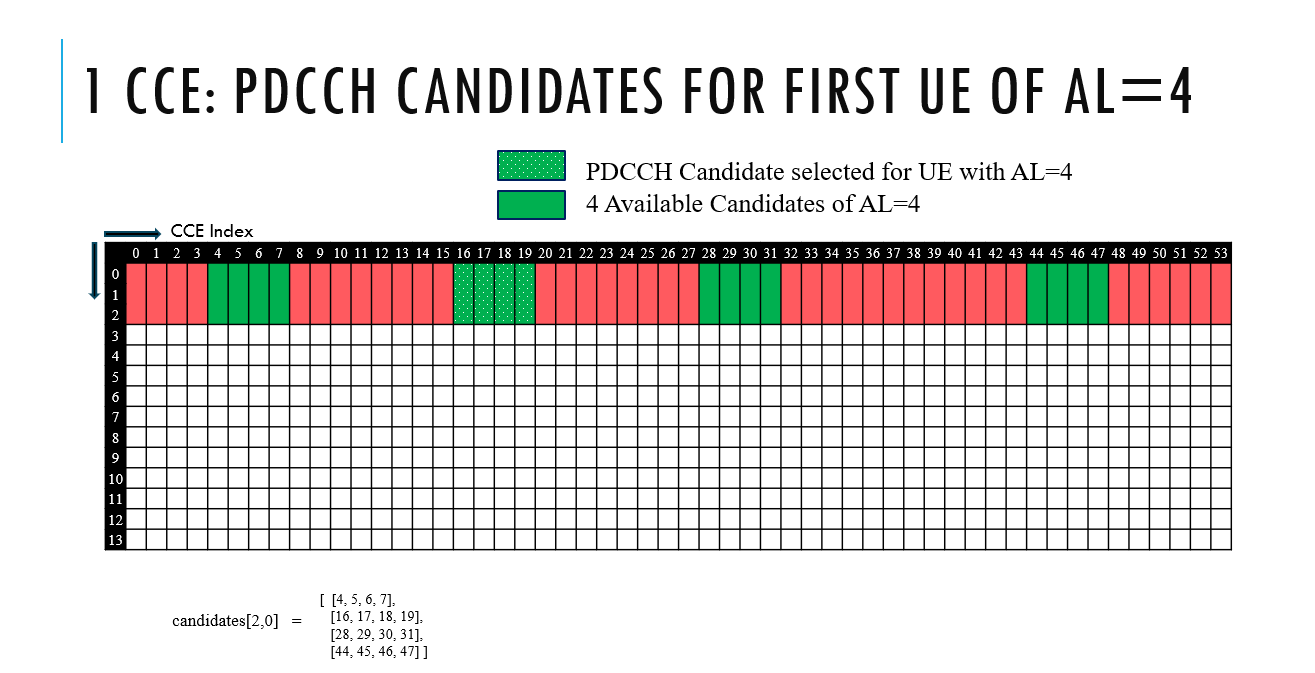
AL 16 candidates are shown below:
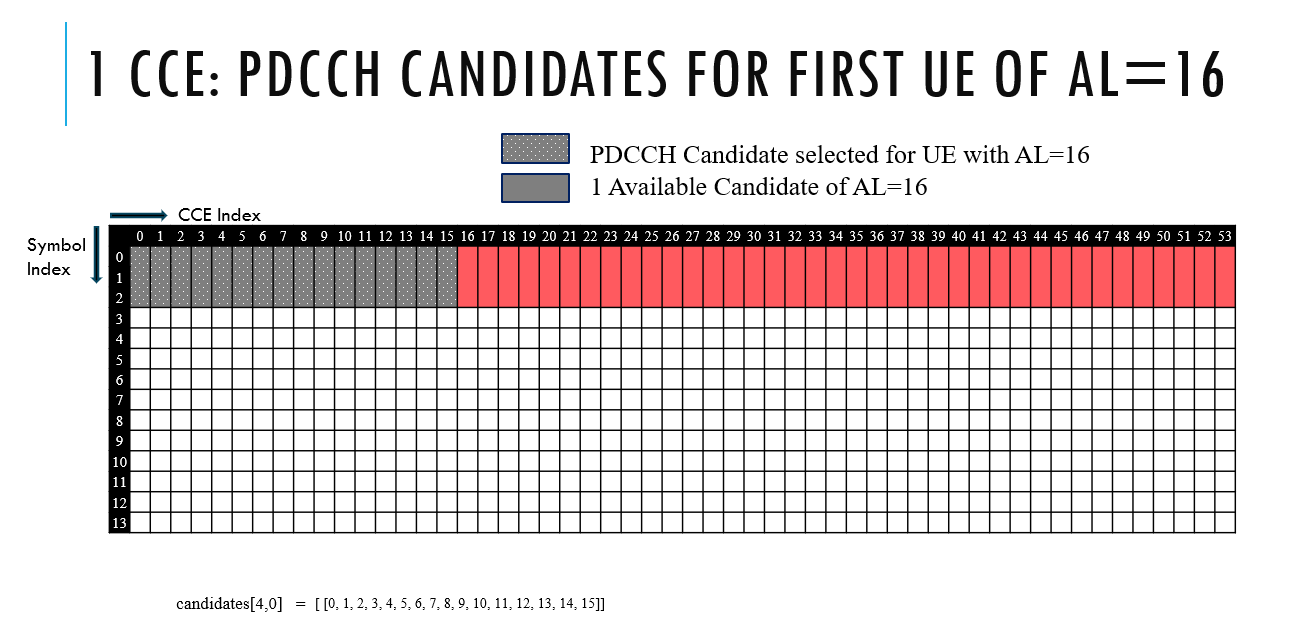
- References:
- [3GPPTS38213PDCCH]
(3GPP TS 38.213 Release 17 V17.1.0); Physical layer procedures for control.